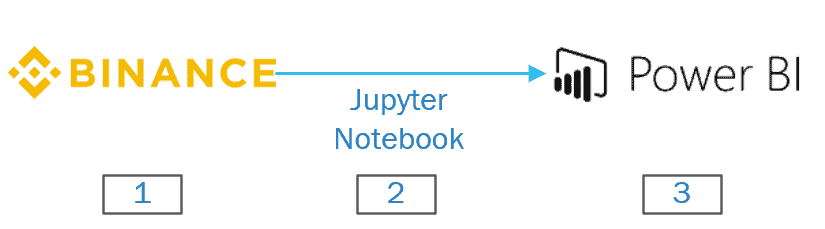
In this notebook, you will extract information about the real-time Bitcoin price in Binance exchange and send it to a Power BI dataset.
You can download the notebook from this link. In upcoming posts, you can take a look at Azure Function instead of Jupyter Notebooks.
In this demo, you will extract the real-time Bitcoin Price using API from cryptocurrency exchange Binance.
Then you’ll take a look at Power BI Rest API with Jupyter Notebooks. Finally, you’ll publish the information into a Power BI dashboard.
Table of Contents
End Goal: display real-time information in Power BI.
#Import Libraries
import requests
import json
from IPython.display import HTML
from time import sleep
from urllib.request import urlopen
from datetime import datetime, timedelta
Get Information from the Binance Exchange using the API
Binance API can be found at https://github.com/binance-exchange/binance-official-api-docs/blob/master/rest-api.md
BinanceResponse = requests.get('https://api.binance.com/api/v3/ticker/price?symbol=BTCUSDT').json()
BinanceConvert = json.dumps(BinanceResponse)
BinanceData = json.loads(BinanceConvert)
print(BinanceData)
Create a dataset with the following columns:
- Timestamp (Datetime)
- Price (Number)
Then, copy the dataset endpoint.
#Paste the report end point copied above
Power_BI_Endpoint = ''
#Repeat for ever
while True:
# The timestamp needs to be formatted correctly
now = datetime.strftime(datetime.now(), "%Y-%m-%dT%H:%M:%SZ")
# Acquire data from the exchange
BinanceResponse = requests.get('https://api.binance.com/api/v3/ticker/price?symbol=BTCUSDT').json()
# Get values from Json response
BinanceConvert = json.dumps(BinanceResponse)
BinanceData = json.loads(BinanceConvert)
Binanceprice=float(BinanceData['price'])
#Format request Json body
Rows = '[{{ "Timestamp": "{0}", "Price": "{1}" }}]'.format(now, Binanceprice)
# make HTTP POST request to Power BI REST API
import urllib.request as ur
req = requests.post(Power_BI_Endpoint, Rows)
# Wait 1 second
sleep(1)
Creating Dashboard and streaming real-time information¶
- Create a Dashboard
- Add Tile
4 Responses
Sandrix
10 . 01 . 2019Good post! Very interesting for beginers.
David Alzamendi
22 . 01 . 2019Thank you!
Jinesh
26 . 05 . 2020Very nice indeed, does this api has param for currency, i.e. NOT US
David Alzamendi
03 . 07 . 2020Hi Jinesh,
I reviewed the API documentation, and I couldn’t find this functionality at this stage.
Regards,
David